My front end development system
development , front-end , symfony2
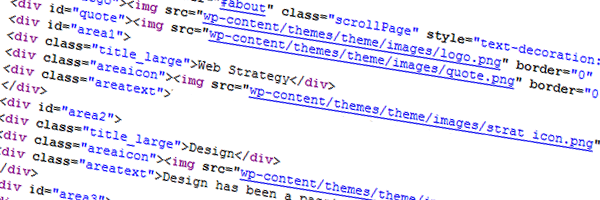
Hi all, I’m preparing a long post in these days, so, to fill this time, I’ll show you how I work with the front end side of a website using the Symfony 2 framework.
I’m not one of those guys who think the core logic behind a website should switch from the server to the client, and I’ll ensure you it will never happen completely, this is why I’m using a PHP framework to explain a faster front end development system.
First of all: Twig. Twig is a template engine that compiles templates down to plain PHP files, as I said many times I think that a web designer shouldn’t work with PHP, they could always try to work with some logics or variables, but they should only show data, not use them.
A naming convention is always a must if you wish to develop something maintainable for a long time, it doesn’t matter what you’re using, if you’re using Twig as the template engine I suggest you to follow the Symfony 2 official file extension system.
While you’re developing a website or a big web application you need to separate roles and let them work indipendently from each other: web designers should work without waiting for the core logic to be ended or all the variables to be passed.
For this reason I suggest to use Faker that generates fake data to fill spaces (for the images use placehold.it). Why is it so useful? Because it can be used with Twig without excessive syntax and it always generates new and random data; for its usage in Symfony 2 add the BazingaFakerBundle and add the following lines to app/config/config_dev.yml
or if you wish to use it in Silex, try my FakerServiceProvider (and tell me if it’s useful).
Before passing out from the server side I want to add some utilities:
- Twig cache extension: I use this extension with the LiipDoctrineCacheBundle, so it’s useless in the development environment, but in production the content is cached and doesn’t need to be recalculated
- Twig Text extension: this is included in the symfony standard edition but, I don’t know why, it’s not included in the Twig extensions and then you should add it to your main bundle in the
Resources/config/services.yml
file - Twig data URI extension: if you wish to hide things like images path in the filesystem or the web URL you can use this extension
- MobileDetectBundle: I think it’s useful if you wish to define something in mobile mode only or in desktop mode only, it adds some useful Twig functions like
is_mobile()
oris_device('iPhone')
After these utilities I suggest you to install two tools, the first one is called FkrCssURLRewriteBundle and it’s used to fix relative paths in assets, the second one is toopay/assetic-minifier because I’ve seen that after cssmin minification, the output isn’t always the same.
This isn’t a bundle but an Assetic extension, so you have to add it from the configuration:
Bower is a powerful JavaScript package manager I use. Create a BowerBundle under your src directory and read this article: blog.ronny.lt/blog/2012/11/05/managing-symfony2-web-assets-with-bower/
If you follow the article indications, you’ll be able to use JavaScript libraries without worrying about the directories or a manual update, everything is maintained using Bower! Remember only to add it to the Assetic configuration under assetic > bundles.
Assetic, as the name suggests, is used to work with assets, in particular to work with meta-languages and with minification, in fact I use it with this configuration
and in Twig files with this code
What’s in this source code? The css_url_rewrite & toopay_cssmin filters are those we defined above, the “?closure” filter means that it will be used only in the production environment and not during development (hopefully, otherwise Google will kick you in few minutes!).
.less files will be elaborated and outputted as CSS in development too, but pay attention: files are not compressed in a single file and converted from less to css as a single file, they are treated individually.
The jshrink tag is useful if you want to compress an inline JavaScript code, you can’t call the Google Closure service (remember that its usage is limited), but you can use this fabolous bundle called SalvaJshrinkBundle that will compress and remove comments from your inline JavaScript, use this tag with the Twig cache extension (inside it) and you’ll gain in performances too!
One last important thing: this entire article was made to encourage front end developers to use some tools and follow some rules while developing. The output isn’t the only important thing. If you need to work with bundles paths in a JavaScript file try the JmikolaJsAssetsHelperBundle, if you receive some not so good variables don’t work on them but request final variables, if you need to work with a release version of APIs, those aren’t available yet, mockup them with apiary.io, don’t try strange ways. Think before acting.